This message, “Type Of Expression Is Ambiguous Without A Type Annotation,” is common in Swift when the compiler cannot determine the exact type of an expression.
In many cases, it means that Swift’s powerful type inference system doesn’t have enough information about the types involved, or the expression is constructed in such a way that multiple interpretations exist.
Over the years, developers have encountered this error in many contexts. Let’s walk through the common causes and how to resolve each one using the insights provided by various experts.
In this article, we will go through the causes and working solutions suggested by experts for this problem, “Type Of Expression Is Ambiguous Without A Type Annotation”.
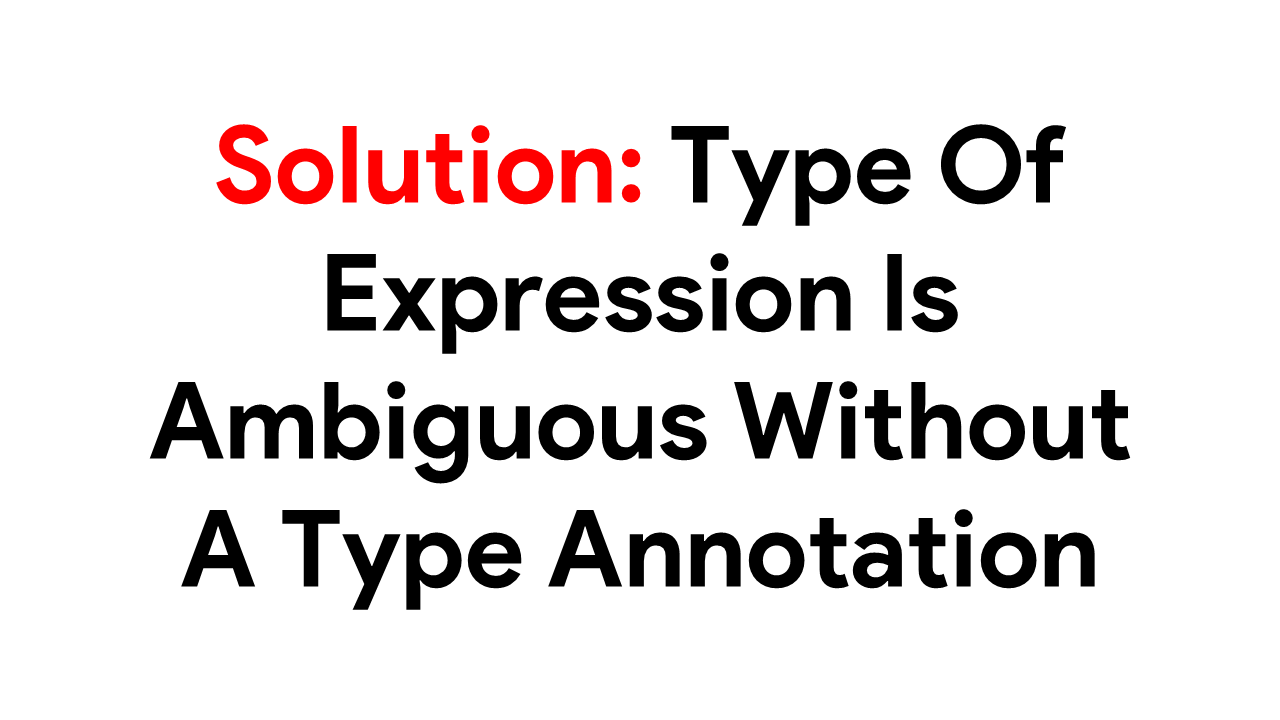
Table Of Contents 👉
1. The Underlying Issue: Ambiguous Type Inference
When Swift sees an expression with mixed literal values or a chain of function calls, it uses context to infer the expected type. If there isn’t enough information, the compiler complains. For example, consider the following mapping:
let imageToDeleteParameters = imagesToDelete.map {
["id": $0.id, "url": $0.url.absoluteString, "_destroy": true]
}
Posts You May Like
Here, the closure returns a dictionary literal with values of different types (an id whose type could be an integer or a string, a URL converted to a string, and a Boolean literal). When Swift cannot decide what type the dictionary it should have, it produces the error.
2. Causes and Solutions Suggested By Experts
Multiple suggestions from the community address this error from different angles. Here are several troubleshooting strategies:
A. Type Mismatch or Incomplete Inference
Mixed Types in Dictionaries or Collections:
When you create a dictionary literal with heterogeneous values (such as String, Bool, numbers, etc.), Swift does not automatically know what the “container” type should be. One common fix is to cast the entire expression to a specific type, such as [String: Any] or [String: AnyObject]. For example:
return [
"title": title,
"is_draft": isDraft,
"difficulty": difficulty,
"duration": duration,
"cost": cost,
"user_id": userId,
"description": description,
"to_sell": toSell,
"images": [imageParameters, imageToDeleteParameters].flatMap { $0 }
] as [String: Any]
This tells the compiler exactly what type of dictionary you expect.
Solution Using Structs:
Instead of building ad-hoc dictionaries where the type cannot be inferred easily, consider creating a data model (a struct or class) that represents the data. This not only provides clarity but also helps you catch mistakes at compile time when a property’s type does not match.
B. Incorrect or Inconsistent Function/Parameter Names
Some cases of this error arise when a function is called with wrong parameter names, or after renaming a variable you forget to update every reference. For example, if the function declaration reads:
func functionWithArguments(argumentName1: Int, argumentName2: Int) { … }
But you call it with:
functionWithArguments(argumentNameWrong: 5, argumentName2: 10)
Even if the error message is not immediately clear, Swift will complain because the compiler cannot match the arguments with any parameter list.
C. Problems with Return Types and Mismatched Types
Another frequent cause is when the type returned by a function does not match what is expected in the context. For example, if you expect a parameter of type URL but pass a String (or vice versa), the compiler might emit this error. In one case, a user had to cast a CGFloat to a Float because a function expected an Objective-C float.
Explicit Type Annotations:
Sometimes, breaking apart a complex expression and annotating intermediate constants with explicit types can help the compiler—and you—to pinpoint where the type mismatch is occurring.
D. Spacing and Syntactic Ambiguities
It might sound trivial, but sometimes stray spaces or misplaced commas can cause the compiler to misinterpret your code. For example:
myFunction(param1: value1 , param2: value2)
versus
myFunction(param1: value1, param2: value2)
Even a misplaced space can confuse the type inference engine.
E. Using Optional Values Without a Default
If you pass optional values (for example, optional strings in a function call) and the function does not expect them—or vice versa—the compiler might not be able to infer a type. In these cases, provide default values with the nil-coalescing operator (??) or adjust the function’s expected parameter types.
For instance, instead of:
router?.pushToDetailsScreen(gitHubRepositoryOwnerName: gitHubRepositoryDetails?[index].owner?.login,
gitHubRepositoryName: gitHubRepositoryDetails?[index].name,
avatarUrl: gitHubRepositoryDetails?[index].owner?.avatar_url)
Use this:
router?.pushToDetailsScreen(
gitHubRepositoryOwnerName: gitHubRepositoryDetails?[index].owner?.login ?? "",
gitHubRepositoryName: gitHubRepositoryDetails?[index].name ?? "",
avatarUrl: gitHubRepositoryDetails?[index].owner?.avatar_url ?? ""
)
Posts You May Like
3. Debugging Strategies
When you encounter this error, try the following steps:
a. Break Down the Expression: Assign intermediate results to constants with explicit types. For example, in a mapping closure, you can write:
let imageToDeleteParameters = imagesToDelete.map { (element: YourType) -> [String: Any] in
return ["id": element.id, "url": element.url.absoluteString, "_destroy": true]
}
This helps the compiler know exactly what type you expect.
b. Check Function Signatures: Ensure that your function names, argument labels, and return types match between declarations and calls.
c. Simplify for Debugging: Replace complex chains (like using flatMap on arrays) temporarily with a simple loop. This can make it easier to see exactly where the type ambiguity is coming from.
d. Consider Using Structs Instead of Dictionaries: As hinted by one of the answers, if you find yourself mapping literal dictionaries with fixed keys, think about creating a model type. This makes your code more type-safe and easier to maintain.
e. Look for Minor Syntactic Issues: Sometimes the error is due to something as simple as an extra space or missing parentheses. Ensure your syntax is precise.
4. Best Practices to Prevent Ambiguity
a. Use Explicit Type Annotations When Needed: If the compiler has difficulty inferring the type, don’t hesitate to annotate your variables or closure parameters explicitly.
b. Prefer Model Types Over Loose Dictionaries: When your data has a known structure, create a struct or class rather than relying on dictionaries.
c. Keep Code Simple: If you are chaining many functional operators (like map, compactMap, or flatMap), consider breaking the chain into smaller, separate statements.
d. Refactor Regularly: After renaming variables or functions, verify that all references match the new names. Use your IDE’s refactoring tools to help with consistency.
Conclusion
The error “Type of expression is ambiguous without more context” usually arises from one or more of the following:
- Mixed types in a literal (especially in dictionaries or arrays)
- Mismatched function or parameter names due to refactoring
- Inaccurate or missing type annotations, especially in closures
- Simple syntactic issues like extra spaces or misplaced commas
By systematically checking your function signatures and providing explicit types, you can resolve ambiguity and help Swift’s compiler understand your intentions, sometimes by switching to model types (structs) instead of generic dictionaries.
Remember, this error isn’t always pointing at the real source of the problem. Breaking complex expressions into smaller parts can often shine a light on the underlying issue.
With practice and by following the best practices described above, you’ll find that resolving these ambiguities becomes second nature.
Posts You May Like