JavaScript Fusker is a pattern‑based scripting technique that dynamically generates sequences of image URLs, allowing developers to enumerate and display large sets of images without manual URL entry.
It has inspired standalone tools and libraries, such as the nektro/fusker image‑extraction tool that fetch and render images on the page from a single URL pattern.
Table Of Contents 👉
Introduction to JavaScript Fusker
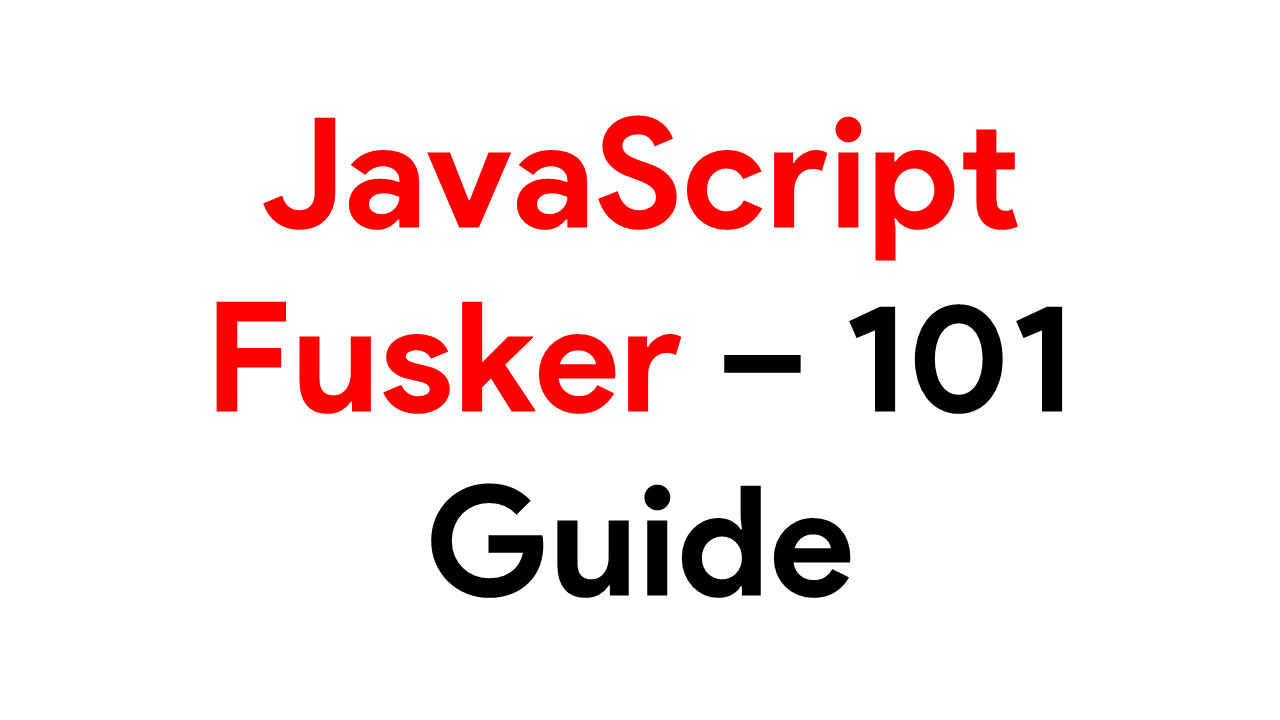
JavaScript Fusker works by defining a URL pattern with embedded ranges, which the script expands into individual URLs for each image in the sequence.
Originally popularized through online demos (e.g., Fusker GUI) for sites with directory browsing disabled, it automates otherwise tedious URL crafting.
While some npm packages named “fusker” serve as application firewalls, image‑enumeration JavaScript Fusker scripts focus on client‑side URL generation and rendering.
Use Cases
- Dynamic Galleries: Quickly build slideshows or thumbnail grids by expanding a single pattern.
- Bulk Downloads: Automate fetching of numbered image series without manual scripting.
- Testing & Prototyping: Generate placeholder image sets for mockups or performance testing.
Getting Started (Beginner)
Basic Pattern Syntax
A javascript Fusker pattern typically uses square brackets with a start and end number:
https://example.com/img[001-010].jpg
Here, [001-010] expands to 001, 002, …, 010, with zero‑padding matching the width of the start value.
Simple Code Example
function generateUrls(pattern) {
return pattern.replace(/\[(\d+)-(\d+)\]/g, (_, start, end) => {
const pad = start.length;
return Array.from({ length: end - start + 1 }, (_, i) =>
String(+start + i).padStart(pad, '0')
).join('|');
}).split('|');
}
// Usage
const urls = generateUrls('https://site.com/photo[1-5].jpg');
console.log(urls);
The generateUrls
function is designed to take a URL pattern that includes a number range, such as 'https://site.com/photo[1-5].jpg'
, and convert it into an array of full URLs by replacing the range with each individual number.
It works by first finding patterns inside square brackets that follow the [start-end]
format using a regular expression.
For example, it detects [1-5]
and captures both the starting number (1
) and ending number (5
). It then creates an array of all numbers between them, including both ends.
If the starting number has leading zeros, it ensures that all generated numbers have the same number of digits using padStart
.
These numbers are joined together with a pipe (|
) symbol to form a single string, and finally, the string is split at each |
to return a complete list of URLs.
So, a pattern like 'https://site.com/photo[1-5].jpg'
becomes an array like ['https://site.com/photo1.jpg', 'https://site.com/photo2.jpg', ..., 'https://site.com/photo5.jpg']
.
Displaying Images
Once you have the URL array, you can append elements or use the Fetch API to load images dynamically. For example:
urls.forEach(src =>
fetch(src)
.then(res => res.blob())
.then(blob => {
const img = document.createElement('img');
img.src = URL.createObjectURL(blob);
document.body.appendChild(img);
})
.catch(() => console.warn(`Failed to load ${src}`))
);
This code takes a list of image URLs stored in the urls
array and tries to fetch each one from the internet. For each URL, it uses the fetch
function to request the image.
Once the image is successfully downloaded, it converts the response into a binary object called a blob. Then, it creates an <img>
element in the document, sets its source to the blob using URL.createObjectURL
, and appends the image to the body of the webpage so it becomes visible.
If any image fails to load, for example, due to a broken link or network issue, it catches the error and logs a warning message to the browser console indicating which image failed.
Alternative Easy Option For Beginner Programmers
The Javascript Fusker tool available on this website is a script designed to display a range of numbered images from a specified URL.
It is particularly useful for websites where directory browsing is disabled, such as image hosting sites.
Key Features:
- Image Range Display: Users can input a URL with a number range (e.g., http://www.example.com/images/image[1-20].jpg) to view multiple images.
- Common Image Names: It works with standard digital camera image names like _IMG_001.jpg and _DSC_001.jpg.
- User Instructions: Users are advised to ensure there are no spaces in the URL for proper loading.
This tool is primarily for educational use.
Other Posts You May Like
Intermediate JavaScript Fusker Techniques
Nested and Multiple Patterns
JavaScript Fusker patterns can nest or combine ranges for complex sequences:
https://site.com/frames[1-3]/layer[a-c].png
You’d recursively expand the first range (1,2,3), then for each result expand [a-c] into a,b,c. A recursive function or multiple .replace() passes can handle this.
Validating and Error Handling
HEAD Requests: To check if an image exists without downloading, send a HEAD request and inspect Content-Type.
Image on error: Dynamically created tags fire an onerror event if loading fails, useful for fallbacks.
Browser Compatibility
Most modern browsers support the Fetch API and padStart. For older environments, consider polyfills for String.prototype.padStart or fallback to XMLHttpRequest for network requests.
Advanced JavaScript Fusker
Building a Custom Fusker Library
- Parser Module: Tokenize patterns ([start-end], nested brackets).
- Expander Module: Recursively build URL lists from parsed tokens.
- Renderer Module: Manage DOM insertion or preloading of images.
- CLI/GUI Interface: Accept patterns via command line or UI form, display progress.
Performance and Concurrency
Uncontrolled parallel fetches can overwhelm servers or hit browser connection limits. Implement a concurrency limiter:
async function fetchWithLimit(urls, limit = 5) {
const iterator = urls.values();
const workers = Array.from({ length: limit }, async () => {
for (const url of iterator) {
try { await fetch(url); }
catch(e) { console.error(`Error loading ${url}`); }
}
});
await Promise.all(workers);
}
This code defines an asynchronous function that fetches multiple URLs but limits how many run at the same time.
It creates a set number of “workers” (default is 5), and each worker takes turns pulling the next URL from the list and fetching it.
All workers share the same iterator, so they divide the work between them. If a fetch fails, it logs an error for that URL.
The function waits for all workers to finish before completing. This helps avoid sending too many requests at once.
Conclusion
JavaScript Fusker empowers developers to automate image‑URL generation, from simple range expansions to building full‑featured libraries with concurrency control and ethical considerations.
Beginners can start with pattern syntax and basic scripts; intermediate users can handle nested patterns and error checking; advanced programmers can architect modular JavaScript Fusker libraries that scale efficiently while respecting server constraints.
Relevant Posts You May Like