In Java applications, you may encounter the error “unable to find valid certification path to requested target” when making HTTPS calls to servers presenting self‑signed certificates or incomplete certificate chains
This article explores the root causes including missing intermediate or root CAs, misconfigured trust stores, and application‑specific keystore settings, and provides exhaustive solutions drawn from community expertise and official documentation.
We cover debugging techniques to trace the SSL handshake, methods to import certificates into Java’s truststore (via keytool, OpenSSL, and custom stores), JVM and IDE configurations (GlassFish, Eclipse, Docker), special‑case workarounds (corporate proxies, AWS Gov RDS), database client settings (sslmode, trustServerCertificate), and best practices to prevent recurring issues.
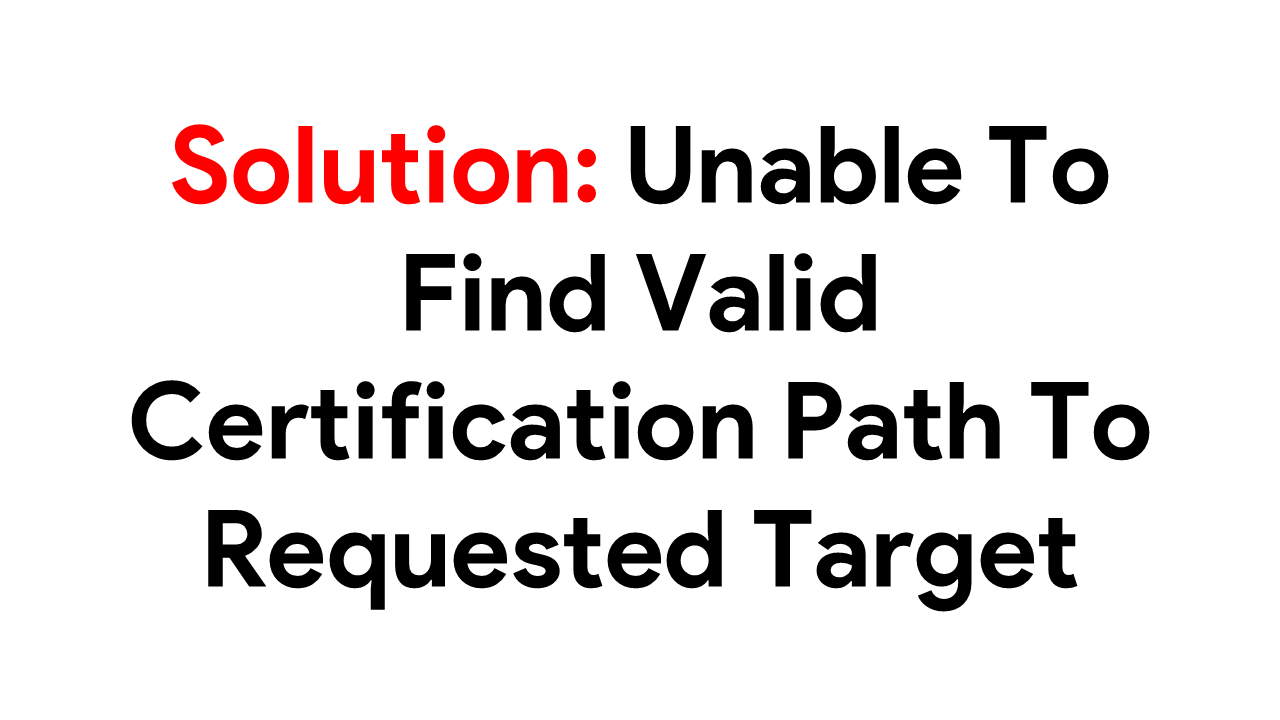
Table Of Contents 👉
Understanding the Error
When a Java client initiates an SSL/TLS handshake, the JDK attempts to build a certification path from the server’s certificate up to a trusted root CA in its truststore.
If any part of the chain is missing or untrusted, the handshake fails with SunCertPathBuilderException: unable to find valid certification path to requested target. This typically occurs with self‑signed certificates or servers that omit intermediate CA certificates.
Keystore vs. Truststore
A keystore holds private keys and the corresponding certificate chains that a Java application uses to identify itself, whereas a truststore contains the public certificates of CAs that the client trusts when verifying a server’s identity.
Confusing the two (for example, importing the server cert into the keystore instead of the truststore) will not resolve the error.
Debugging SSL Handshakes
Enable detailed SSL debugging to trace validation steps and pinpoint missing certificates:
java -Djavax.net.debug=all \
-Djavax.net.ssl.trustStore=/path/to/truststore \
-Djavax.net.debug=ssl,handshake,keymanager,trustmanager \
-jar YourApp.jar
This prints every handshake message, certificate validation, and trust manager decision to help isolate the failure point.
On PaaS platforms like Heroku, ensure your dyno’s JDK truststore includes the necessary CAs, or overlay a custom cacerts file as documented in the Heroku Dev Center.
Other Posts You May Like
Importing Certificates
4.1 Default CACerts Import via Keytool
The simplest fix is to import the server’s certificate into the JRE’s default cacerts truststore:
keytool -importcert \
-trustcacerts \
-alias myserver \
-file server.cer \
-keystore $JAVA_HOME/jre/lib/security/cacerts \
-storepass changeit
This approach works for standalone Java apps and application servers reading the default truststore.
4.2 Creating a Custom Truststore
For isolation or multi‑environment setups, create a separate truststore:
keytool -importcert \
-alias myserver \
-file server.cer \
-keystore myTrustStore.jks \
-storepass changeit
Then instruct the JVM to use it:
-Djavax.net.ssl.trustStore=/path/to/myTrustStore.jks \
-Djavax.net.ssl.trustStorePassword=changeit
This prevents mixing test certs with the system CAs.
4.3 OpenSSL + Keytool for Self‑Signed Certs
Extract the certificate directly from the server and import it:
echo -n | openssl s_client -connect host:443 \
| openssl x509 > extracted.cert
keytool -importcert \
-alias extracted \
-file extracted.cert \
-keystore $JAVA_HOME/jre/lib/security/cacerts \
-storepass changeit
This ensures you capture the exact cert the server presents.
4.4 Importing Full Certificate Chains
When intermediate CAs are involved, fetch the entire chain:
openssl s_client -showcerts -connect host:443 </dev/null \
| sed -n '/BEGIN/,/END/p' \
> fullchain.pem
# Split and import each cert in fullchain.pem
csplit -sk fullchain.pem '/-BEGIN CERTIFICATE-/' '{*}'
for cert in xx*; do
alias=$(openssl x509 -noout -subject -in $cert | sed -n 's/.*CN=//p')
keytool -importcert \
-alias "$alias" \
-file $cert \
-keystore cacerts \
-storepass changeit \
-noprompt
done
This procedure handles all levels of the certificate hierarchy.
Configuring SSL Properties
5.1 JVM Arguments (trustStore & trustStorePassword)
Always supply both the store path and password when deviating from defaults:
-Djavax.net.ssl.trustStore=/custom/path/truststore.jks \
-Djavax.net.ssl.trustStorePassword=changeit
Omitting the password or using a relative path may lead to silent failures where the cert isn’t actually loaded.
5.2 IDE & Container Environments
Eclipse/STS: These IDEs bundle their own JRE. Import certs into the IDE’s JRE keystore, not the system JDK.
Docker: Link the container’s cacerts to the host system’s certificate bundle or copy a prepared cacerts into the image:
RUN rm -f /opt/jdk/jre/lib/security/cacerts \
&& ln -s /etc/ssl/certs/java/cacerts \
/opt/jdk/jre/lib/security/cacerts
Relevant Posts You May Like
Special‑Case Workarounds
6.1 Corporate Proxies & Security Brokers
Security products like NetSkope can inject their own certs into the chain, breaking validation. Temporarily disabling the proxy or importing its root cert resolves the issue
6.2 Database Client SSL Modes
For JDBC connections, database drivers often expose SSL parameters:
PostgreSQL: set sslmode=require or verify-full as needed.
MSSQL: add trustServerCertificate=true to the connection string to bypass chain validation when appropriate.
Cloudflare Origin Pulls: choose the correct encryption mode (Full, Full (strict), etc.) in your CDN or proxy settings.
6.3 AWS Gov RDS Certificates
When connecting to AWS GovCloud RDS, download the special CA bundle and import all contained certs into your truststore:
curl -o gov-rds.pem \
https://s3.us-gov-west-1.amazonaws.com/rds-downloads/rds-combined-ca-us-gov-bundle.pem
# split and import as shown above
After updating the truststore, restart your Java process to apply changes.
Best Practices
- Always validate the full certificate chain (use openssl s_client -showcerts).
- Avoid modifying the default cacerts in production; prefer custom truststores.
- Automate certificate updates in CI/CD to prevent expired or rotated certs from causing outages.
- When possible, rely on a well‑maintained CA hierarchy rather than self‑signed certs.
- Use strict SSL/TLS modes in proxies or CDNs to enforce end‑to‑end encryption
Conclusion
By understanding the distinction between keystores and truststores, leveraging detailed SSL debugging, and applying the appropriate certificate import and JVM configuration techniques, you can resolve SunCertPathBuilderException errors across diverse Java environments.
Whether you’re importing a single self‑signed cert, handling corporate proxies, or configuring database SSL modes, the solutions outlined here cover every high‑voted approach from the community.
Consistent certificate management and thorough testing are key to preventing future SSL path validation failures.
Relevant Posts You May Like